In some cases, you might need to validate a form field on the front end, ensuring a user doesn’t input invalid data.
In this tutorial, without relying on server-side validation we will be preventing a negative numbers in Textbox Fields.
Instead of implementing a full-fledged validation plugin, you can use a simple JavaScript code snippet. This is where the JavaScript section in Formea Form Builder comes in handy.
Example
Try entering a value less than zero in the input field below, the field should reset to empty and an error message prompt.
Where to find the element Alias and ID
In order to manipulate the field value, we need to know the element alias and ID.
Navigate to
Formea Form Builder > Elements > [THE_TARGETED_ELEMENT].
See screenshot to
locate the element alias and ID.
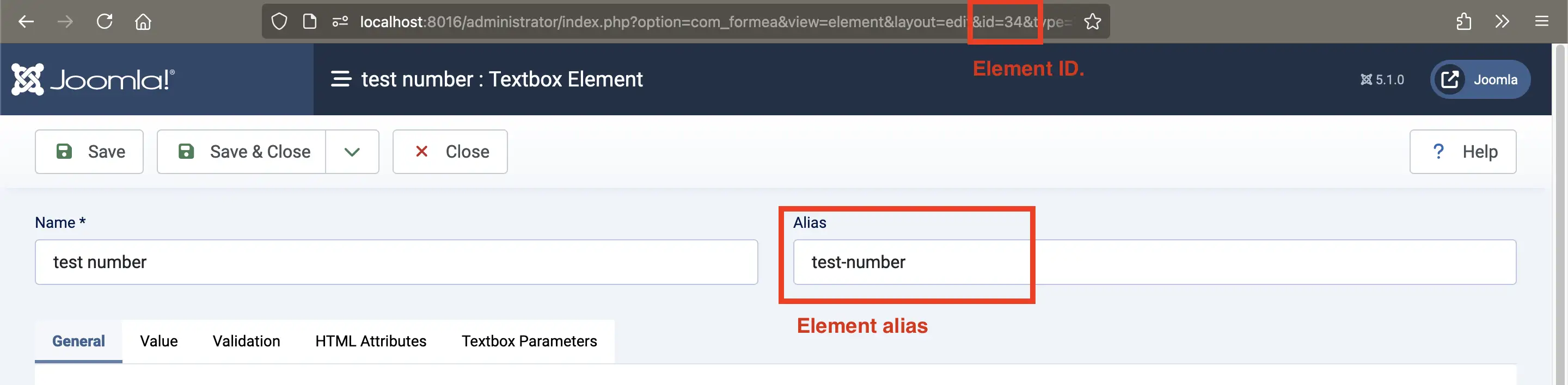
Steps to Implement JavaScript for Field Manipulation
-
Navigate to Your Form in Formea:
Go to Joomla! Administrator > Components > Formea Form Builder > Forms > [YOUR_FORM_NAME].
-
Access the JavaScript Section:
- Click on the "CSS/JavaScript" tab.
- Scroll to the "JavaScript Declaration" section where you'll add your custom JavaScript.
-
Add the JavaScript Code: Below is a sample JavaScript code that prevents negative numbers from being entered in a specified field. Replace
"MY_ELEMENT_ALIAS"
with the field's alias and"MY_ELEMENT_ID"
with the field's ID. -
Save your form:
Click "Save" or "Save & Close" to store your changes.
JavaScript Code
This is an example of code designed to detect an input value and determine whether it’s a positive or negative number.
For better readability and flexibility—allowing for reuse or modification to accommodate other elements or fields—we will extract the logic into functions.
setUpValidation:
This function sets the input’s minimum value and attaches an event listener to monitor changes. It calls updateValidationState
to update the input's appearance based on its validity.
updateValidationState:
This function handles toggling classes for valid/invalid feedback and updates the inner text of the error message div. The classes are based on a template that uses the Bootstrap CSS framework, like Joomla’s default Cassiopeia template. You may need to adjust the classes to match your own template.
Feel free to modify this according to your requirements.